- Published on
Architecture to build UI framework using Sass
7 min read
- Authors
- Name
- Abdelfatah Ashour
- @abdoashour07
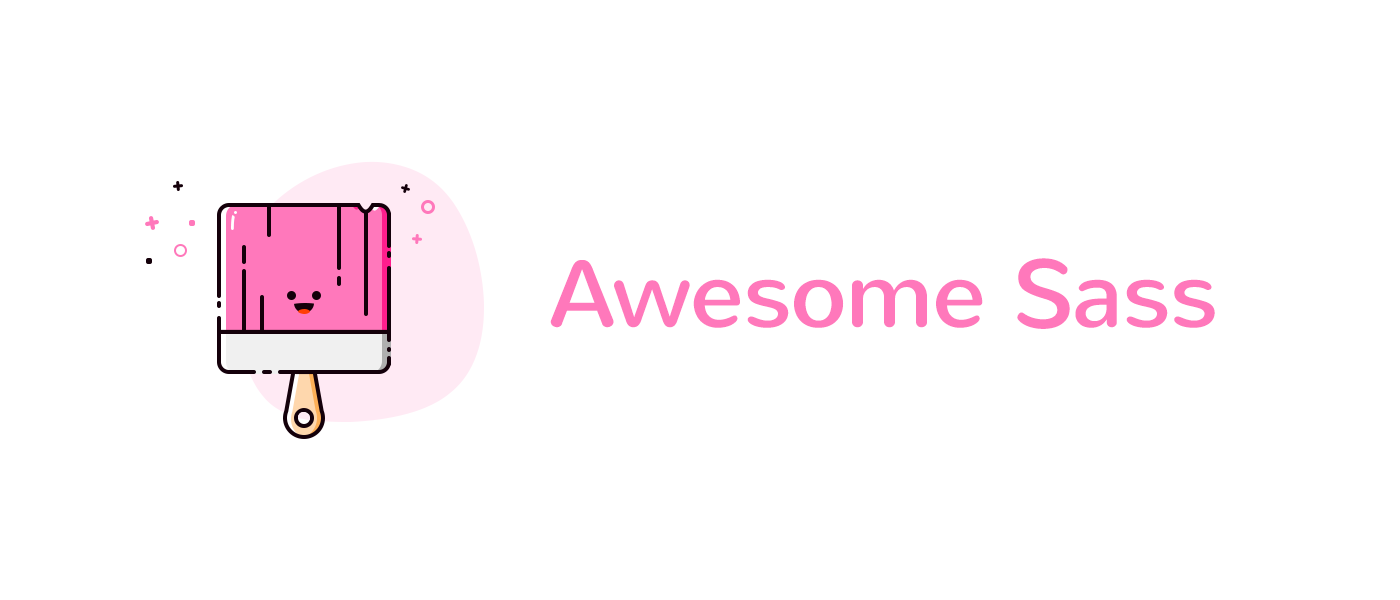
Table of Contents
step by step guide to build UI framework using Sass:
Here is one possible architecture for building a UI framework using Sass:
Define a component library: Start by defining a library of reusable components that can be used throughout your application. These components should be designed to be flexible and customizable, allowing developers to easily modify their appearance and behavior to fit their specific needs.
Create a base stylesheet: Develop a base stylesheet that defines the basic styles for your application. This stylesheet should include global styles like typography, color schemes, and layout rules that apply to all components in your library.
Develop a Sass mixin library: Create a library of Sass mixins that can be used to easily create new styles for your components. These mixins should be designed to be flexible and modular, allowing developers to easily modify them as needed.
Use Sass variables: Leverage Sass variables to make your stylesheets more maintainable and easier to modify. For example, you could define a variable for your primary color scheme and then use that variable throughout your stylesheets to ensure consistency.
Utilize inheritance and nesting: Take advantage of Sass's inheritance and nesting features to simplify your stylesheets and make them easier to read and understand. Use inheritance to define shared styles between components, and use nesting to encapsulate styles for specific components.
Use partials: Divide your stylesheets into partials to make them more modular and easier to manage. For example, you could create a partial for each component in your library and then include those partials as needed in your main stylesheet.
Define a build process: Define a build process that compiles your Sass code into a single, minified CSS file for use in your application. You may also want to include features like autoprefixing and linting to ensure that your stylesheets are optimized and error-free.
By following these steps, you can create a flexible, modular UI framework using Sass that can be easily customized and extended to fit your specific needs.
Example:
Here is a possible architecture for building a UI framework using Sass, along with some examples:
Define a component library: Start by defining a library of reusable components that can be used throughout your application. For example, you could define a button component like this:
Create a base stylesheet: Develop a base stylesheet that defines the basic styles for your application. For example, you could define your typography and color schemes like this:
Develop a Sass mixin library: Create a library of Sass mixins that can be used to easily create new styles for your components. For example, you could create a mixin to handle responsive styles like this:
Use Sass variables: Leverage Sass variables to make your stylesheets more maintainable and easier to modify. For example, you could define a variable for your primary color scheme and then use that variable throughout your stylesheets to ensure consistency.
Utilize inheritance and nesting: Take advantage of Sass's inheritance and nesting features to simplify your stylesheets and make them easier to read and understand. For example, you could define a base component class and then extend that class for specific components like this:
Use partials: Divide your stylesheets into partials to make them more modular and easier to manage. For example, you could create a partial
Define a build process: Define a build process that compiles your Sass code into a single, minified CSS file for use in your application. You can use tools like Gulp, Grunt, or Webpack to automate this process.
// Button component
.btn {
display: inline-block;
padding: 8px 16px;
font-size: 16px;
font-weight: bold;
text-align: center;
border-radius: 4px;
cursor: pointer;
background-color: $primary-color;
color: #fff;
transition: background-color 0.3s ease;
&:hover {
background-color: darken($primary-color, 10%);
}
&:active {
background-color: darken($primary-color, 20%);
}
&:focus {
outline: none;
box-shadow: 0 0 0 3px rgba(0, 0, 0, 0.2);
}
}
// Typography
$font-family: Arial, sans-serif;
$font-size-base: 16px;
$line-height-base: 1.5;
body {
font-family: $font-family;
font-size: $font-size-base;
line-height: $line-height-base;
}
h1, h2, h3, h4, h5, h6 {
font-weight: bold;
}
p {
margin-bottom: 1em;
}
// Color scheme
$primary-color: #007bff;
$secondary-color: #6c757d;
$success-color: #28a745;
$warning-color: #ffc107;
$error-color: #dc3545;
// Responsive mixin
$screen-sm: 576px;
$screen-md: 768px;
$screen-lg: 992px;
$screen-xl: 1200px;
@mixin respond-to-sm {
@media (min-width: $screen-sm) {
@content;
}
}
@mixin respond-to-md {
@media (min-width: $screen-md) {
@content;
}
}
@mixin respond-to-lg {
@media (min-width: $screen-lg) {
@content;
}
}
@mixin respond-to-xl {
@media (min-width: $screen-xl) {
@content;
}
}
// Base component class
.component {
margin-bottom: 1em;
}
// Button component
.btn {
@extend .component;
}
// Input component
.input {
@extend .component;
}
for each component in your library like this:
// _buttons.scss partial
@import "variables";
// Button component
.btn {
...
}
// _inputs.scss partial
@import "variables";
// Input component
.input {
...
}
Conclusion:
By following these steps, you can create a flexible, modular UI framework using Sass that can be easily customized and extended to fit your specific needs.